Get started with the SDK
This article provides steps to include the SDK in your Android app's code so that your app can initialize the SDK and start using the SDK's features.
Wait. Are you ready for this part?
We hope that you could set up the SDK in your app's project. If not, then we suggest that you set up the SDK in your project and then come back here to include it in your code.
We'll wait. Take your time. No pressure. ☺️
Initialize the SDK
To get started, the host app should initialize the blueshift sdk with a valid configuration.
Mandatory configurations
To initialize the SDK with the minimum requirements, add the following code to your app's Application
file's onCreate()
method.
Following are the minimum requirements for the SDK to get initialized.
- App icon: The app icon resource id is required to show the icon on the push notification if not explicitly provided.
- Event API key: This value is required to authenticate the events. You could find it here in the dashboard.
Configuration configuration = new Configuration();
// == Mandatory Settings ==
configuration.setAppIcon(R.mipmap.ic_launcher);
configuration.setApiKey("BLUESHIFT_EVENT_API_KEY");
Blueshift.getInstance(this).initialize(configuration);
Event API Key
Ensure that you set the API key before proceeding. The integration fails if you don't specify the API key here.
Application file
The SDK must be initialized from your Application file's
onCreate()
method. Failure to do so may result in push notifications not being displayed when your app is in the killed state.
Application file? Huh?
When you create a project in the Android Studio for your app, you define the application file in the
AndroidManifest.xml
file of the project. When the app is built, the code from this file is the first component that gets started when a user runs your app on a device. For example, in the sample Blueshift Reads Android app that we provide for reference, you can see theAndroidManifest.xml
file. In this file, you can see that the application class is mapped to theReadsApplication.java
file.In this example, the
onCreate()
function resides in theReadsApplication.java
file.
Optional configurations
Following are optional configs. If you are going to use the SDK with only push and events (with default settings), you may skip to the next section. Curious minds can read ahead.
Data center region
The SDK version 3.2.4 and above lets you choose the Blueshift data center region during SDK initialization. You can choose between the US and the EU. The default value is the US.
configuration.setRegion(BlueshiftRegion.EU);
Push notification
The push notifications are enabled by default. But if the host app does not want to display push notifications from blueshift, then you could turn off the push notifications using the below method.
configuration.setPushEnabled(false);
This is purely a client-side setting. The blueshift server won't know that push is disabled if you turn it off using this method. To fully opt-out from the push, please refer to this document.
Push notification style
The SDK lets you customize the style of the notification by changing the icons and color.
// Provide appropriate icon resources to change the big and small icons.
// Default: App icon.
configuration.setLargeIconResId(R.drawable.notification_big_icon);
configuration.setSmallIconResId(R.drawable.notification_small_icon);
// The color of notification. This requires Android 21 or higher.
// Default: System default.
int color = ContextCompat.getColor(getApplicationContext(), R.color.notification_color);
configuration.setNotificationColor(color);
// The theme for Dialog type notifications. (Deprecated.)
// Default: System default.
configuration.setDialogTheme(R.style.dialog_theme);
Push notification channel (Android 8 or higher)
Android Oreo onwards, the notification framework requires a notification channel to be defined when you create a notification. The Android OS will skip the notification if a channel is not provided.
The below methods will let you provide a default value for notification channel id, name, and description. These values will be used when the push notification payload does not contain the value for notification channel id, name, and description.
// Default: "bsft_channel_General"
configuration.setDefaultNotificationChannelId("app_channel_id_default");
// Default: "General"
configuration.setDefaultNotificationChannelName("app_channel_name_default");
// Default: None. This is only set if present in payload or config object.
configuration.setDefaultNotificationChannelDescription("app_channel_description_default");
Deeplinks
When the user clicks on the push notification, the SDK will open the host app's LAUNCHER/MAIN activity and deliver the URL as a string extra inside the bundle with the key "deep_link_url".
To read this value you could make use of the below code snippet.
String deepLinkURL = getIntent().getStringExtra(RichPushConstants.EXTRA_DEEP_LINK_URL);
InApp Messages
In-app messages are not enabled by default. To enable them, call the below two methods.
configuration.setInAppEnabled(true);
// This consent is required to show HTML in-app messages that had JavaScript in them.
configuration.setJavaScriptForInAppWebViewEnabled(true);
Interval
When more than one in-app messages are available for display, the default time interval between two in-app messages is 1 minute. This is the case when the user remains on the same page for 1 minute after dismissing an in-app message. This time interval is configurable with the configuration object as mentioned below.
configuration.setInAppInterval(seconds);
Background fetching
The SDK can fetch the in-app message content from API in the background when a silent push message is received from Blueshift. This is enabled by default in SDK v3.1.1 and higher. If you wish to disable it, you could do it as follows.
configuration.setInAppBackgroundFetchEnabled(false);
Manual Mode
The SDK does the in-app fetching and displaying automatically. If the host app needs full control over this, they can enable manual mode when they initialize the SDK.
When enabled, the SDK
- will NOT fetch in-app message on app start.
- will NOT show in-app message automatically (at any cost).
- will only fetch and store the in-app messages when a silent push is received.
- will only display in-app message when user invokes the
Blueshift.getInstance(this).displayInAppMessages();
method.
configuration.setInAppManualTriggerEnabled(true);
Mobile Inbox
A mobile inbox contains the in-app messages that are directly delivered to the inbox in a non-intrusive way or displayed in the app and delivered to the inbox. Users will see the messages in their inbox until the message expires or until they delete them after reading them.
You could enable mobile inbox using the following configuration
configuration.setInboxEnabled(true);
To know more about this feature, check out the documentation.
When you enable mobile inbox, the inapp messaging feature will be enabled automatically on the SDK side.
DeviceID source
If you are an existing Blueshift customer and have not touched this part yet, we want you to exercise caution if you choose a new device ID source. If you have anonymous users (users who use your app without signing in), choosing a new device ID source will delete all the data that we collect.
The SDK is flexible enough to let the host app decide what needs to be collected as "device_id". The default option is INSTANCE_ID_PKG_NAME. The other possible options and their example values are as follows.
GUID
The SDK can generate a random GUID and use it as the device_id
. SDK can cache this value to be used across different app sessions. If required, the host app can reset this value by using a helper method provided in the SDK.
Min SDK version: 3.0.9 | Example: 6ca4a79a-ee53-4372-bb81-165ade978e1f
configuration.setDeviceIdSource(Blueshift.DeviceIdSource.GUID);
Reset GUID
GUID is the ONLY resettable device ID provided by the SDK. To reset, please use the method given below.
Blueshift.resetDeviceId(context);
We recommend using GUID as the
device_id
since it is convenient to reset it when user logs out.
INSTANCE_ID
The SDK can collect the Firebase Instance ID and use it as the device_id
.
Min SDK version: 3.0.9 | Example: d-FEG4gYT6ygf63wEVu4nP
configuration.setDeviceIdSource(Blueshift.DeviceIdSource.INSTANCE_ID);
INSTANCE_ID_PKG_NAME (Default since v3.2.6)
The SDK can collect the Firebase Instance ID and the package name and form a combination value to be used as device_id
.
Min SDK version: 3.1.1 | Example: d-FEG4gYT6ygf63wEVu4nP:com.blueshift.reads
configuration.setDeviceIdSource(Blueshift.DeviceIdSource.INSTANCE_ID_PKG_NAME);
CUSTOM
Most of the use cases will be covered using the above options. But, sometimes the host app may come with a custom-made device_id. In that case, the SDK can even accept that value and use it in all the events.
Min SDK version: 3.1.4
configuration.setDeviceIdSource(Blueshift.DeviceIdSource.CUSTOM);
String custom_id = getMyCustomDeviceId();
configuration.setCustomDeviceId(custom_id);
ADVERTISING_ID
Deprecated
Deprecated since v3.2.6
The SDK can collect the Android Ad ID as device_id
automatically.
Min SDK version: 3.0.9 | Example: 65fb85f5-cf16-4616-8b2e-a55c1434fda9
configuration.setDeviceIdSource(Blueshift.DeviceIdSource.ADVERTISING_ID);
ADVERTISING_ID_PKG_NAME
Deprecated
Deprecated since v3.2.6
The SDK can collect the Android Ad ID and the package name and form a combination value to be used as device_id
.
Min SDK version: 3.1.1 | Example: 65fb85f5-cf16-4616-8b2e-a55c1434fda9:com.blueshift.reads
configuration.setDeviceIdSource(Blueshift.DeviceIdSource.ADVERTISING_ID_PKG_NAME);
Get the current device ID
In some use cases, the host app would want to know the device ID being used by the Blueshift SDK, in such cases, the host app could call the following method to get the same.
DeviceUtils.getDeviceId(context);
Please note that the above method will return null
, when the device_id
is not generated at the time of the call. In such cases, we should retry.
Batched events time interval
The SDK lets you send low-priority events in batches (of 100 events) to Blueshift's bulk event API at a regular interval of 30min by default. This interval is configurable using the below-mentioned method.
/*
* This is the time interval used for batching events which are then sent to
* Blueshift using the bulk events api call. It defaults to 30 min if not set.
*
* It is highly recommended to use one of the following for API < 19 devices.
* AlarmManager.INTERVAL_FIFTEEN_MINUTES
* AlarmManager.INTERVAL_HALF_HOUR
* AlarmManager.INTERVAL_HOUR
* AlarmManager.INTERVAL_HALF_DAY
* AlarmManager.INTERVAL_DAY
*/
configuration.setBatchInterval(timeInMilliSeconds);
The SDK can send events in both realtime and batches. The last argument of every event tracking method (canBatchThisEvent) defines this behavior. To send events in batches, set it to
true
.Ex: Blueshift.getInstance(mContext).trackEvent("event_name", params, true)
We highly recommend the use of real-time events as batched events won't give expected results if you plan to use them in campaigns.
Override Job ID
Our SDK uses JobScheduler for intelligent scheduling of background jobs on Android 21 or higher.
If you use the same in your apps and come across a conflict in Job IDs used, you could override the same as follows.
// for bulk event triggering service's job id
configuration.setBulkEventsJobId(newId);
// for network change listener's job id.
configuration.setNetworkChangeListenerJobId(newId);
Automatic app_open event
The SDK can automatically fire an app_open
event of every app start (from the killed state). This can be enabled using the below method.
configuration.setEnableAutoAppOpenFiring(true);
From v3.1.9 onwards, the default behavior is to fire one automatic app_open
event in 24 hours. To change this time interval, please make use of the below method.
configuration.setAutoAppOpenInterval(seconds);
Encrypt the customer information
The user information is stored as plain text by default inside the shared_pref folder of the app. From v3.5.0 and above, the SDK supports storing the user information in an encrypted form. This setting can be enabled using the saveUserInfoAsEncrypted
flag as mentioned below.
configuration.setSaveUserInfoAsEncrypted(true);
Few warnings to consider, if you plan to start using this feature.
- The preference file should not be backed up with Auto Backup. When restoring the file it is likely the key used to encrypt it will no longer be present. You should exclude the preference file "com.blueshift.encrypted.preferences" from the backup using backup rules.
- Once the encryption is enabled, we can not go back and use the
SharedPreference
(unencrypted) implementation with another app update. It can cause issues. - EncryptedSharedPreferences requires a minimum Android API version of 23. As a result, the library's minSdkVersion has been bumped to 23.
Setting Customer Information
Use the UserInfo
class to send the customer's details to Blueshift. If the values are set after the customer signs in, they will be used to build the parameters for all the events going forward.
Here is an example of how to set email
and customer_id
after a customer signs in:
UserInfo userInfo = UserInfo.getInstance(context);
// Setting "email"
userInfo.setEmail("[email protected]");
// Setting "customer_id"
userInfo.setRetailerCustomerId("customer:1234");
// Setting additional key-value pairs
// Here is an example of signing events.
HashMap<String,Object> extras = new HashMap<>();
extras.put("verification_key", "[email protected]");
extras.put("event_signature", "e88f85c920f59002409a4c71fde4c0c08ccb0ea464a0e0c96b46508ef0afd27d");
userInfo.setDetails(extras);
// It is important to save the instance once an updation is made on UserInfo
userInfo.save(context);
// Use this method to clear existing user info after a logout.
userInfo.clear(context);
Exclude SDK from ProGuard
If you have enabled ProGuard in your project, please exclude the SDK by adding the following lines to your proguard-rules.pro
file.
-keep class com.blueshift.** { *; }
-dontwarn com.blueshift.**
Logging
If you are using the SDK version 3.1.1 or higher, no Log messages will be printed. To enable logging, add the following line before initializing the SDK.
BlueshiftLogger.setLogLevel(BlueshiftLogger.VERBOSE);
Disable tracking
Min SDK version 3.1.10
You can now disable sending custom events, push & in-app metrics to the Blueshift. Note that, disabling will drop all the pending events which are yet to send to Blueshift.
The tracking is enabled by default. To disable call,
Blueshift.setTrackingEnabled(context, false);
Messaging opt-out
Push Notifications
You can use Blueshift app preferences to opt-out of push messages that we send from our platform. You can use this functionality if you don't want push messages from our platform to show up on your app.
BlueshiftAppPreferences.getInstance(context).setEnablePush(true);
BlueshiftAppPreferences.getInstance(context).save(context);
InApp Messages
On SDK version 3.2.0 or higher, you can use Blueshift app preferences to opt-out of in-app messages that we send from our platform. You can use this functionality if you don't want in-app messages from our platform to show up on your app.
BlueshiftAppPreferences.getInstance(context).setEnableInApp(true);
BlueshiftAppPreferences.getInstance(context).save(context);
Ensure that you trigger an identify event so that we don't push messages to the customer that you specify.
Request push notification permission (Android 13)
Requires SDK version 3.3.0 and above.
To get explicit consent from users to receive push notifications from your app before you send push notifications to them, you can launch the Blueshift SDK’s push permission request workflow by using the blueshift://req-push-permission deep link in your in-app message.
In your in-app template, set the context for sending the push notifications.
- For the “Allow” button, use the URL blueshift://req-push-permission to launch the Blueshift SDK’s push permission request workflow.
- For the “Deny” button, use the URL blueshift://dismiss. This will help you track the number of users who opted out of push notifications.
When a user clicks an in-app notification button to "Allow" push notifications, a push permission dialog is displayed and the user can grant the permission. If the user had already granted push permission, the dialog is not displayed.
If the push notification permission is denied twice, a dialog is displayed with a message asking the user to go to the app settings to enable push notifications.
Refer to the following screenshots to understand better the above behavior.
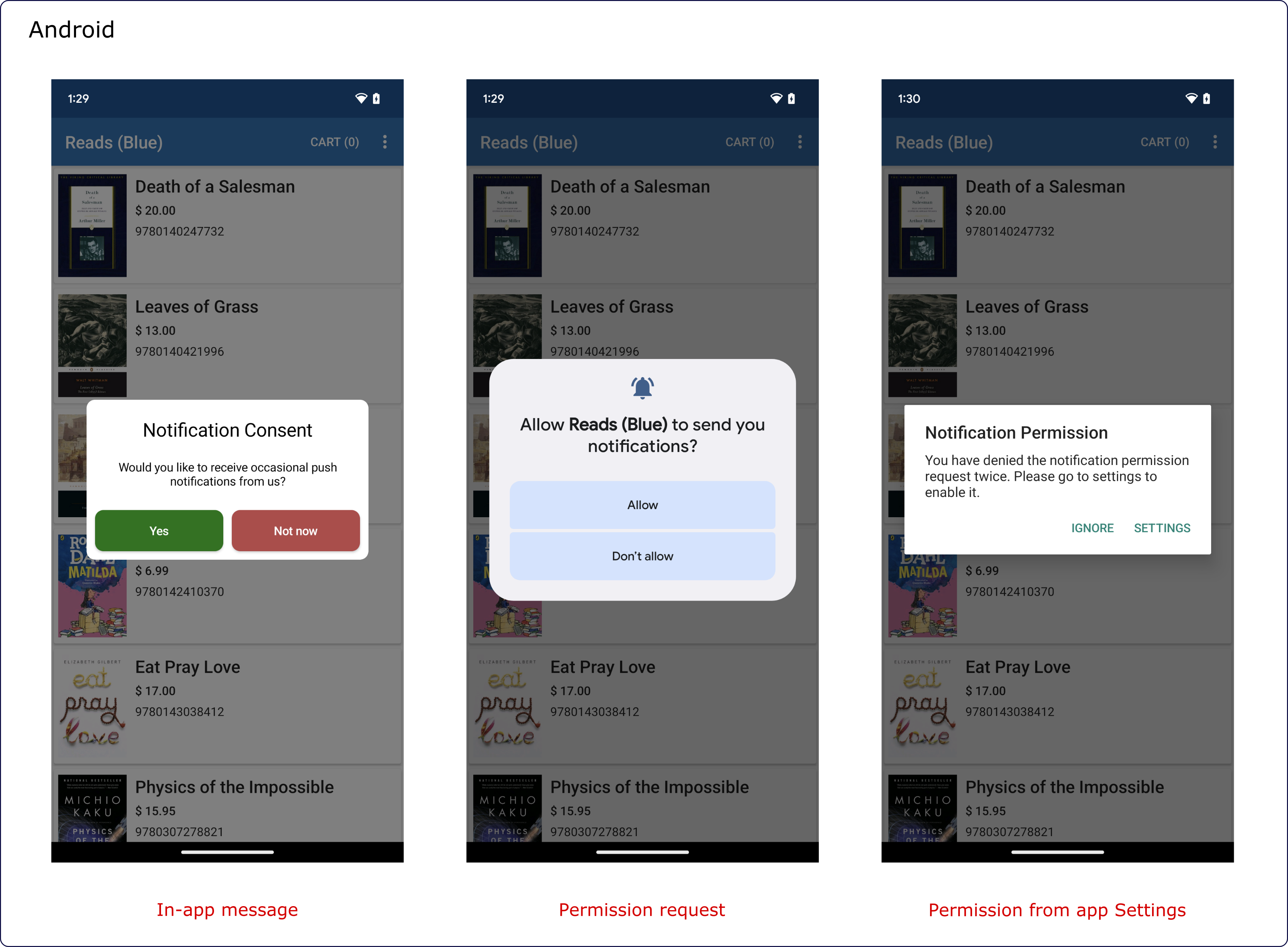
Point out Blueshift's messages
If you send messages to your customers in addition to the ones from the Blueshift platform, you can use the following helper methods to point out which messages come from our platform:
BlueshiftUtils.isBlueshiftPushMessage(intent);
BlueshiftUtils.isBlueshiftPushMessage(remoteMessage);
Test the SDK integration
These are some of the guidelines to verify the correctness of the SDK integration. The most important thing is to ensure that the app is working as it was before integrating with the SDK.
There are 4 components to verifying the integration:
- SDK is initialized correctly
Ensure that certain events are launched correctly from the app, such asidentify
,click
, andapp_open
. - Dashboard
Confirm that the events show up correctly in the activity of a user on the Blueshift dashboard. - Notifications
Use the push studio editor to create different types of push messages and ensure that the notifications render correctly on devices. - Campaigns
Create a test campaign with the test devices and run a campaign against them to ensure that you are getting stats.
That's it! You are all set to start using the SDK!
Updated 5 days ago
Now that you are familiar with our SDK, you can start using it to track events on your app. For more information, see: