Get started with the SDK
This article provides steps to include the SDK in your app's code so that the app can initialize the SDK and start using the SDK's features.
Wait. Are you ready for this part?
Have you set up the SDK and added all the files into your Xcode project? If not, then complete the steps to set up the SDK in your project before you start including the SDK in your code.
We'll wait. Take your time. No pressure. ☺️
Permissions required
Blueshift requires the following permissions in your project to send notifications to the end user and collect events from your app:
- To send push notifications, enable Push Notifications in the Xcode project of your app.
- To send silent push notifications, enable Remote notifications background mode in the Xcode project of your app.
After adding the Push Notifications capability and enabling Remote notifications background mode, it should look like this:
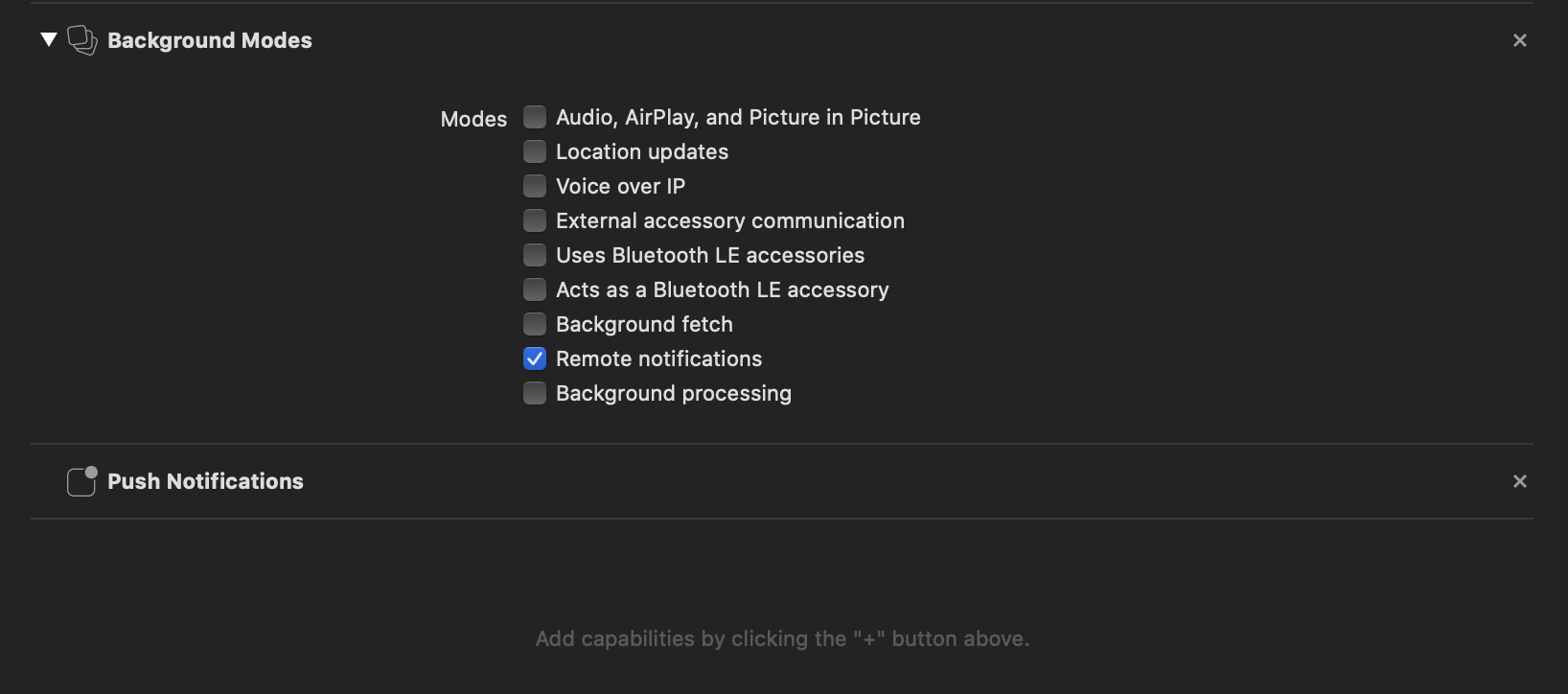
Initialize the SDK
To get started, include the SDK's header file in the AppDelegate.h
or AppDelegate.swift
file of the app's Xcode project.
If the programming language is Objective-C, include the SDK's header in AppDelegate.h
:
<BlueShift-iOS-SDK/BlueShift.h>
@interface AppDelegate : UIResponder <UIApplicationDelegate>
If the programming language is Objective-C, add the following code in the AppDelegate.m
file. If it's Swift, import the SDK in AppDelegate.swift
and add the following code:
import BlueShift_iOS_SDK
class AppDelegate: UIResponder, UIApplicationDelegate
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Obtain an instance of BlueShiftConfig
let config: BlueShiftConfig = BlueShiftConfig()
// Required: Set the api Key for the config
config.apiKey = "Your API Key here"
// Required for EU region: set the region to EU
// Optional for US region: default is US if not set
config.region = .US // Change to .EU only if using EU region
// Optional: Set user notification delegate. This delegate will be used while
// registering for remote notifications. In case you don't set it up,
// SDK will set its own delegate.
config.userNotificationDelegate = self
// Optional: Set AppGroupId only if you are using the Carousel push notifications.
config.appGroupID = "Your App Group ID here"
// Optional: From v2.2.0, Set custom authorization options
config.customAuthorizationOptions = [.alert, .badge, .sound, .providesAppNotificationSettings]
// Optional: From v2.2.0, Set custom categories
config.customCategories = getCustomeCategories()
// Optional: Set Batch upload interval in seconds.
// If you do not add below line, SDK by default sets it to
// 300 seconds.
BlueShiftBatchUploadConfig.sharedInstance().batchUploadTimer = 60
// Optional: SDK uses IDFV by default if you do not
// include the following line of code.For more information,
// see: https://developer.blueshift.com/docs/include-configure-initialize-the-ios-sdk-in-the-app#specify-the-device-id-source
config.blueshiftDeviceIdSource = .idfvBundleID
// Optional: Set debug true to see Blueshift SDK info and api logs, by default its set as false.
config.debug = true
// Optional: Change the SDK core data files location only if needed. The default location is the Document directory.
config.sdkCoreDataFilesLocation = .libraryDirectory
// Set the applications launch Options for SDK to track
config.applicationLaunchOptions = launchOptions
// Initialize the SDK with config and integrate the SDK manually.
BlueShift.initWithConfiguration(config)
return true
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Obtain an instance of BlueShiftConfig.
BlueShiftConfig *config = [BlueShiftConfig config];
// Required: Set the api Key for the config.
[config setApiKey:@"Your API Key here"];
// Required for EU region: set the region to EU
// Optional for US region: default is US if not set
[config setRegion:BlueshiftRegionUS]; // Change to BlueshiftRegionEU for EU region
// Optional: Set user notification delegate. This delegate will be used while registering for remote notifications. In case you don't set it up, SDK will set its own delegate.
[config setUserNotificationDelegate:self];
// Required from v2.1.7: Set AppGroupId to improve push notification delivery and click tracking
[config setAppGroupID:@"Your App Group ID here"];
// Optional: From v2.2.0, Set custom authorization options
config.customAuthorizationOptions = UNAuthorizationOptionAlert| UNAuthorizationOptionSound| UNAuthorizationOptionBadge| UNAuthorizationStatusProvisional;
// Optional: From v2.2.0, Set custom categories
config.customCategories = [self getCustomeCategories];
// Optional: Set Batch upload interval in seconds.
// If you do not add below line, SDK by default sets it to
// 300 seconds.
[[BlueShiftBatchUploadConfig sharedInstance] setBatchUploadTimer:60.0];
// Optional: SDK uses IDFV by default if you do not
// include the following line of code.For more information, see:
// https://developer.blueshift.com/docs/include-configure-initialize-the-ios-sdk-in-the-app#specify-the-device-id-source
[config setBlueshiftDeviceIdSource: BlueshiftDeviceIdSourceIDFVBundleID];
// Optional: Set debug true to see Blueshift SDK info and api logs, by default its set as false.
[config setDebug:YES];
// Optional: Change the SDK core data files location only if needed. The default location is the Document directory.
[config setSdkCoreDataFilesLocation:BlueshiftFilesLocationLibraryDirectory];
// Set the applications launch Options for SDK to track.
[config setApplicationLaunchOptions:launchOptions];
// Initialize the configuration
[BlueShift initWithConfiguration:config];
return YES;
}.
If you look at the code, you can see that we are creating a config
class of the type BlueShiftConfig
. This is an important class that corresponds to the SDK's configuration. This class specifies the values our SDK requires to work properly. To initialize the SDK successfully, your app should create and pass a valid instance of this class to the SDK.
SDK Config values
The values that are required to configure the SDK are:
API key
The SDK uses the event API key to connect to our platform and send data the SDK collects from your app to our platform. This is a mandatory field, and ensure that you provide it here.
You can get the event API key from the API Keys tab in Account page of the Blueshift app. Provide the Event API Key for this setting.
Blueshift Region
iOS SDK v2.2.3 onwards, you can set the region in the SDK config. Blueshift supports two regions: US
and EU
.
You must set the region explicitly if your account is in the EU region.
If the region is not set, the SDK defaults to the US
region, which is valid only for US accounts.
Failure to set the region for EU accounts will result in API calls failing silently.
userNotificationDelegate
The SDK uses this delegate while registering for the remote notifications and iOS gives a callback to the implemented userNotification
delegate methods when push notification action/event happens.
If you skip setting this delegate, SDK will create its own delegate and use it while registering for remote notifications. All the userNotification
push notification action/event will then go to SDK's delegate.
appGroupID
AppGroupId is mandatory to set only if you are using the Carousel push notifications. The click tracking for the carousel push notification will not work correctly if you do not set it.
Debug flag
iOS SDK v2.1.7 onwards, you can see the SDK logs. The SDK logs are divided into 4 categories:
- Errors
- Exceptions
- Info
- API call info
Errors
and Exceptions
are printed by default. To enable info
and API call info
logs, set config.debug = true
during SDK initialization.
DeviceIdSource
SDK sets IDFV as the deviceIdSource
by default, if you don't set it manually. If you have multiple apps under one Blueshift account, then we recommend setting it to the idfvBundleID
option.
SDK Also provides UUID
and custom
options as device id sources. Refer this section for more details.
Launch options
The SDK uses this option to track if the app is launched from a push notification.
CustomAuthorizationOptions & CustomCategories
You can now set the custom push notification authorization options and custom push notification categories to SDK. SDK will use them while registering for remote notifications. You can refer to this section for more details.
sdkCoreDataFilesLocation
SDK creates core data files by default in the app's Document
directory. If your app supports sharing the Document
directory or Document browser
, then you can change this location to the Library
directory, so that the SDK files won't show up in the app's Document
directory.
Here, SDK takes care of moving existing core data files(if present) from the Document
directory to the Library
directory.
You can set the Library files location as -config.sdkCoreDataFilesLocation = .libraryDirectory
.
Changing files location from the Library
directory to the Document
directory is not recommended. SDK does not take care of moving the files from the Library
directory to the Documents
directory.
batchUploadTimer
The SDK uses this option to upload the data to Blueshift at this specified intervals in batches.
Make sure you do not set the batchUploadTimer
to 0 seconds. Find more about the batch uploads here.
Note
The API key is a mandatory value that you need to specify before initializing the SDK. The rest of the methods are optional, so you can use them to customize the SDK and use its features based on your requirements.
Auto-integration
Call method initWithConfigurationAndAutoIntegrate
to integrate the SDK automatically using given config. SDK uses method swizzling to auto integrate the iOS callbacks. If you opt to auto integrate the SDK, then you can skip Configure AppDelegate for push notifications and section as SDK takes care of handling it automatically.
// Initialize the configuration required to be executed on the main thread
BlueShift.initWithConfigurationAndAutoIntegrate(config)
// Initialize the configuration.
[BlueShift initWithConfiguration:config];
// Auto Integration
[BlueShift autoIntegration];
Configure AppDelegate for push notifications
In addition to configuring push notifications, add the following to the AppDelegate
file of your app's Xcode project. Configuring the remoteNotification
and userNotificationCenter
delegate methods will
- Share the device token received from the OS with SDK
- Share the push and silent notification payload to SDK
- Share the push notification click response to SDK
SDK automatically fires an identify
call when it receives a device token for the very first time and when the device token gets changed.
In case if you have integrated push notifications from multiple sources, you can identify if a push notification is sent from Blueshift using the SDK helper method. You can refer to this document for more details.
//configure remote notifications
extension AppDelegate {
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
BlueShift.sharedInstance()?.appDelegate?.register(forRemoteNotification: deviceToken)
}
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
BlueShift.sharedInstance()?.appDelegate?.failedToRegisterForRemoteNotificationWithError(error)
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
BlueShift.sharedInstance()?.appDelegate?.handleRemoteNotification(userInfo, for: application, fetchCompletionHandler: completionHandler)
}
}
//configure UserNotificationCenter
extension AppDelegate: UNUserNotificationCenterDelegate {
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
BlueShift.sharedInstance()?.userNotificationDelegate?.handleUserNotification(center, didReceive: response, withCompletionHandler: completionHandler)
}
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
BlueShift.sharedInstance()?.userNotificationDelegate?.handle(center, willPresent: notification, withCompletionHandler: completionHandler)
}
}
//configure remote notifications
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(nonnull NSData *)deviceToken {
[[BlueShift sharedInstance].appDelegate registerForRemoteNotification:deviceToken];
}
- (void)application:(UIApplication*)application didFailToRegisterForRemoteNotificationsWithError:(NSError*)error {
[[BlueShift sharedInstance].appDelegate failedToRegisterForRemoteNotificationWithError:error];
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult result))handler {
[[BlueShift sharedInstance].appDelegate handleRemoteNotification:userInfo forApplication:application fetchCompletionHandler:handler];
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(nonnull NSDictionary *)userInfo {
[[BlueShift sharedInstance].appDelegate application:application handleRemoteNotification:userInfo];
}
- (void)application:(UIApplication *) application handleActionWithIdentifier: (NSString *) identifier forRemoteNotification: (NSDictionary *) notification completionHandler: (void (^)()) completionHandler {
[[BlueShift sharedInstance].appDelegate handleActionWithIdentifier:identifier forRemoteNotification:notification completionHandler:completionHandler];
}
//configure UserNotificationCenter
-(void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions options))completionHandler{
[[BlueShift sharedInstance].userNotificationDelegate handleUserNotificationCenter:center willPresentNotification:notification withCompletionHandler:^(UNNotificationPresentationOptions options) {
completionHandler(options);
}];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
[[BlueShift sharedInstance].userNotificationDelegate handleUserNotification:center didReceiveNotificationResponse:response withCompletionHandler:^{
completionHandler();
}];
}
Setting user info
Use the BlueShiftUserInfo
class to send the user details to Blueshift. SDK will add values saved in the BlueShiftUserInfo
to each event and send it to the Blueshift server. The user-specific details can be saved when the user is logged in to the app.
In order to associate the events to the correct user, there are three primary identifiers used to identify a user uniquely.
- DeviceID - This will be auto-generated by SDK and sent to the Blueshift server as
device_id
attribute as part of every event. You can refer to this document to find more on the device Id types supported by the SDK. - EmailId - Email id needs to be set to
BlueShiftUserInfo.sharedInstance()?.email
variable whenever it changes and this will be sent to Blueshift server as part of every event. - CustomerId - Customer id needs to be set to
BlueShiftUserInfo.sharedInstance()?.retailerCustomerID
SDK variable whenever it changes and this will be sent to Blueshift server as part of every event.
Here is an example of how to set customer_id
, email
, and additional user information after a user signs in:
// On sign in complete
BlueShiftUserInfo.sharedInstance()?.email = "User Email ID"
BlueShiftUserInfo.sharedInstance()?.retailerCustomerID = "User Customer ID"
//Set other user info as below. This info will be used for running
//personalized campaigns
BlueShiftUserInfo.sharedInstance()?.firstName = "add first name"
BlueShiftUserInfo.sharedInstance()?.lastName = "add last name"
BlueShiftUserInfo.sharedInstance()?.gender = "add gender"
BlueShiftUserInfo.sharedInstance()?.dateOfBirth = "add DOB"
//From SDK v2.1.17, you can set custom user attributes to the extras dictionary
BlueShiftUserInfo.sharedInstance()?.extras = ["userType":"Premium","subExpiryDate":"15-10-2021"]
// It is important to save the information after updating it
BlueShiftUserInfo.sharedInstance()?.save()
// On sign in complete
[[BlueShiftUserInfo sharedInstance] setEmail:@"User Email ID"];
[[BlueShiftUserInfo sharedInstance] setRetailerCustomerID: "User Customer ID"];
//Set other user info as below. This info will be used for running personlised campaigns
[[BlueShiftUserInfo sharedInstance] setFirstName: @"add first name"];
[[BlueShiftUserInfo sharedInstance] setLastName: @"add last name" ];
[[BlueShiftUserInfo sharedInstance] setGender: @"add gender"];
[[BlueShiftUserInfo sharedInstance] setDateOfBirth: @"add DOB"];
//From SDK v2.1.17, you can set custom user attributes to the extras dictionary
[[BlueShiftUserInfo sharedInstance] setExtras:@{@"userType":@"Premium",@"subExpiryDate":@"15-10-2021"}];
// It is important to save the information after updating it
[[BlueShiftUserInfo sharedUserInfo] save];
Reset user info
When you set the user info to SDK, it is also important to reset the user info. On logout, the same user info which was set for the logged-in user needs to be cleared. you can clear the current user info as below:
BlueShiftUserInfo.removeCurrentUserInfo()
[BlueShiftUserInfo removeCurrentUserInfo];
Register the user with Blueshift
As soon as the user information is saved, run the identifyUser
event. The identifyUser
event is used to identify the end-user who signed into the app. The primary identifier used to identify a user is device_id
that is passed to the Blueshift. The device_id
is set during the SDK initialization, and it is automatically sent to Blueshift. In addition to sending the device_id
, we recommend that you set the email
and customer_id
of the user. This ensures that we can still attribute the events to the user and generate recommendations for the user if the device_id
resets.
After sending the identify event, you will be able to find the user profile on the Blueshift Dashboard -> Customer Data -> Attributes using the email id or customer id.
Identify event is responsible to update the data for the user profile on the Blueshift server. Whenever any BlueshiftUserInfo
data gets changed, we recommend you send an identify event to update those changes on the Blueshift server.
//Identify user using email id
BlueShift.sharedInstance().identifyUser(withEmail: "User Email ID", andDetails: nil, canBatchThisEvent: false)
//Identify user with customer id, make sure you have set the retailerCustomerID
//in UserInfo as below before calling identify.
BlueShiftUserInfo.sharedInstance()?.retailerCustomerID = "User Customer ID"
BlueShiftUserInfo.sharedInstance()?.save()
BlueShift.sharedInstance().identifyUser(withDetails:nil, canBatchThisEvent: false)
//Identify user with device id if email and customer id is not present
BlueShift.sharedInstance().identifyUser(withDetails:nil, canBatchThisEvent: false)
//Identify user with custom details
let details = ["name": "Ross Geller", "profession":"paleontologist"]
BlueShift.sharedInstance().identifyUser(withDetails:details, canBatchThisEvent: false)
//Identify user using email id
[[BlueShift sharedInstance] identifyUserWithEmail:@"User Email ID" andDetails:nil canBatchThisEvent:NO];
//Identify user with customer id, make sure you have set the retailerCustomerID in UserInfo as below before calling identify.
[[BlueShiftUserInfo sharedInstance] setRetailerCustomerID: "User Customer ID"];
[[BlueShiftUserInfo sharedInstance] save];
[[BlueShift sharedInstance] identifyUserWithDetails:nil canBatchThisEvent:NO];
//Identify user with device id if email and customer id is not present
[[BlueShift sharedInstance] identifyUserWithDetails:nil canBatchThisEvent:NO];
//Identify user with custom details
NSDictionary *details = @{ @"name":@"Ross Geller",
@"profession":@"paleontologist" }
[[BlueShift sharedInstance] identifyUserWithDetails:details canBatchThisEvent:NO];
Batch uploads
The SDK uploads the data that it collects on your app either in batches or in real time. If the device is offline and the app triggers the SDK events, all these events will be cached and sent to the server in batches of 100 when the device goes online.
The last argument in any SDK event-specific method decides whether an event is sent either in real-time or in a batch.
BlueShift.sharedInstance().trackEvent(forEventName: "testing swift", canBatchThisEvent: false)
You can see that the last parameter we use is canBatchThisEvent
and it is set to false
. In this case, the event is sent to us in real-time. Based on your need, you can either send the events in real time or as batch events.
Deep links on iOS
The Blueshift iOS SDK supports deep links on push, in-app, and inbox messages. If a deep-link URL is present in the push, in-app, and inbox message payload, the Blueshift SDK triggers AppDelegate application:openURL:options:
on notification click/tap action and delivers the deep link there.
The host app will need to write some business logic in order to process the delivered deep link and navigate to the respective screen inside the app.
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey : Any] = [:]) -> Bool {
// Handle deep link url
return true;
}
- (BOOL)application:(UIApplication *)app openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey, id> *)options {
// Handle deep link url
return YES;
}
Advanced configurations
Delay Push Notification Permission
If you don't want the push notification permission to be displayed on the app launch, you can customize it to display it later after sign up/sign in. To do that you need to set the config.enablePushNotification
as false while initializing the SDK.
//Disable push notifications in the SDK configuration in appDelegate to
//delay the Push notification permission
config.enablePushNotification = false
//Disable push notifications in the SDK configuration in appDelegate to delay the Push notification permission
[config setEnablePushNotification:NO];
Now, you need to enable the push notifications and call the registration for remote notification where you want to display the Push notification permission dialog.
//Enable the push and call registerForNotification to show Push permission
BlueShift.sharedInstance()?.config.enablePushNotification = true
BlueShift.sharedInstance()?.appDelegate.registerForNotification()
//Enable the push and call registerForNotification to show Push permission
[[[BlueShift sharedInstance]config] setEnablePushNotification: YES];
[[BlueShift sharedInstance].appDelegate registerForNotification];
Specify the device ID source
You can use any device id source from below mentioned options.
- UUID : Randomly generated Unique id - Recommended for multi-app usecase. (supported from SDK v2.1.3)
- IDFV : Apple Identifier for vendor (supported from SDK v2.1.3)
- IDFVBundleID : Combination of IDFV and Bundle ID - Recommended for multi-app usecase. (supported from SDK v2.1.3)
- custom : Custom device id provided by the app (supported from SDK v2.1.6)
By default, the SDK picks IDFV
as device id if you do not set it explicitly in the config. However, you can customize the SDK so that it picks UUID
or IDFVBundleID
instead.
If you are an existing Blueshift client, and have not touched this part yet, we want you to exercise caution if you choose a new device ID source. If you have anonymous users (users who use your app without signing in), choosing a new device ID source will delete all the data that we collect.
Use the following method to specify the device ID source you want the SDK to pick.
UUID
This type provides a randomly generated unique device id, which is resettable based on your use case.
Important
We recommend you to use UUID type device id as it generates a new device id per app installation and it is resettable if required.
You may want to reset the device id on logout to track the logout user's activity separately using a new id. If you don't reset the device ID, the logged-out user's activity will continue to be tracked using the same device ID and will be linked to the same logged-out user.
// Add below line to select UUID device id type to SDK.
// The device id for this type looks like c2a766bd-bb45-486f-88ae-b939c4534864
config.blueshiftDeviceIdSource = .UUID
// Add below line to select UUID device id type to SDK.
// The device id for this type looks like c2a766bd-bb45-486f-88ae-b939c4534864
[config setBlueshiftDeviceIdSource: BlueshiftDeviceIdSourceUUID];
Reset the UUID type Device Id
From SDK v2.2.5, You can reset the UUID
type of device id. In order to make use of this function, you need to choose the UUID
type as your deviceIdSource. Set the device id source in SDK config as config.blueshiftDeviceIdSource = .UUID
.
Calling this method will reset the existing UUID
device id, and SDK will generate a new device id. SDK will also send an identify
event to update the device to Blueshift. This method will not work for device id type IDFV
or IDFV:BundleId
.
// Reset device id, so that SDK will create a new device id.
BlueShiftDeviceData.current().resetDeviceUUID()
// Reset device id, so that SDK will create a new device id.
[[BlueShiftDeviceData currentDeviceData] resetDeviceUUID];
IDFV
SDK uses the Apple identifier for the vendor as the default device id for the SDK. This device id value is same for the apps that come from the same vendor running on the same device. The IDFV can be returned the same even after app reinstallation if you have another app installed from the same vendor on your device. You can check more on this here.
// This type is default device id type set by SDK. The IDFV device id looks like - 24c4bd97-5cb3-458a-aa41-e0106770cf07
config.blueshiftDeviceIdSource = .IDFV
// This type is default device id type set by SDK. The IDFV device id looks like - 24c4bd97-5cb3-458a-aa41-e0106770cf0
[config setBlueshiftDeviceIdSource: BlueshiftDeviceIdSourceIDFV];
IDFVBundleId
This device id type is a combination of IDFV and the bundle id.
// Add below line to select idfvBundleId device id type to SDK.
// The device id for this type looks like 24c4bd97-5cb3-458a-aa41-e0106770cf07:com.blueshift.reads
config.blueshiftDeviceIdSource = .idfvBundleID
// Add below line to select idfvBundleId device id type to SDK.
// The device id for this type looks like 24c4bd97-5cb3-458a-aa41-e0106770cf07:com.blueshift.reads
[config setBlueshiftDeviceIdSource: BlueshiftDeviceIdSourceIDFVBundleID];
Custom Device ID
If the above device id sources don't meet the requirements, from SDK version 2.1.6 and higher, Blueshift iOS SDK can accept a custom device ID from the host app. This let's you reuse the device_id in use.
Important
Please make sure you set a valid device-id during the SDK initialization. SDK does not store the custom device id and depends on providing it at the time of SDK initialization. The provided device id should not be empty or null and should not contain spaces/special characters.
To set your own device ID, choose the blueshiftDeviceIdSource
as custom
and then set the customDeviceId
value.
//Set deviceIDSource as custom
config.blueshiftDeviceIdSource = .custom
//Set the custom device id value
config.customDeviceId = "SET CUSTOM DEVICE ID HERE"
//Set deviceIDSource as custom
[config setBlueshiftDeviceIdSource: BlueshiftDeviceIdSourceCustom];
//Set the custom device id value
[config setCustomDeviceId:@"SET CUSTOM DEVICE ID HERE"];
Access the device ID used by SDK
You can access the device id value used by the SDK by calling the following SDK method.
let deviceId = BlueShiftDeviceData.current().deviceUUID
NSString* deviceId = [BlueShiftDeviceData currentDeviceData].deviceUUID;
Messaging opt-out
The Messaging out-out are of two types,
- Device level opt-out
- User level opt-out
Device level opt-out
This is recommended approach as this opt-out is very specific to a device. If the user or app opts out of device level messaging, Blueshift will only disable it for a that individual device and you can still send the messages to other devices associated with the user.
Device level Opt out of push messages
From iOS SDK v2.1.8, you can use Blueshift app preferences to opt-out of push notifications that we send from our platform. You can use this functionality if you don't want push notifications from our platform to show up on your app for a device. After modifying the value, fire an identify event to update the data on the Blueshift server.
// Device level Opt out of push messages
BlueShiftAppData.current()?.enablePush = false
// Device level Opt out of push messages
[[BlueShiftAppData currentAppData]setEnablePush:NO];
Device level Opt out of in-app messages
From iOS SDK v2.1.17, you can use Blueshift app preferences to opt-out of in-app notifications that we send from our platform. You can use this functionality if you don't want in-app messages from our platform to show up on your app for a device. After modifying the value, fire an identify event to update the data on the Blueshift server.
// Device level Opt out of in-app messages
BlueShiftAppData.current()?.enableInApp = false
// Device level Opt out of in-app messages
[[BlueShiftAppData currentAppData]setEnableInApp:NO];
Ensure that you trigger an identify event so that we don't send push/in-app notifications to the customer that you specify.
In order to start receiving the push or in-app notifications, make sure you re-enable the
enablePush
/enableInApp
flag and fire identify event.
User level opt-out
This opt-out is specific to a user. If a user opts-out of user level messaging, then Blueshift will not send any campaigns to all the devices associated with that user. The user level opt-out needs to be used with care as it can disable all the devices associated with the user from receiving the messages.
User level Opt out of push messages
You can make use of BlueshiftUserInfo
preference unsubscribed
or send unsubscribed_push
as true
from the identify event.
If you use the BlueshiftUserInfo
preference method, then make sure you fire identify event after that to update the server.
// User level Opt out of push messages
// Use BlueshiftUserInfo preference as true or false
BlueShiftUserInfo.sharedInstance()?.unsubscribed = true
BlueShift.sharedInstance()?.identifyUser(withDetails: nil, canBatchThisEvent: false)
// ------------- OR ---------------------------------
// Fire identify event with unsubscribed_push as true or false
BlueShift.sharedInstance()?.identifyUser(withDetails: ["unsubscribed_push":true], canBatchThisEvent: false)
// User level Opt out of push messages
// Use BlueshiftUserInfo preference as YES or NO
[[BlueShiftUserInfo sharedInstance] setUnsubscribed:@YES];
[[BlueShift sharedInstance] identifyUserWithDetails:nil canBatchThisEvent:NO];
// ------------- OR ---------------------------------
// Fire identify event with unsubscribed_push as YES or NO
[[BlueShift sharedInstance] identifyUserWithDetails:@{@"unsubscribed_push":@YES} canBatchThisEvent:NO];
User level Opt out of in-app messages
There is no BlueshiftUserInfo
preference available for in-app out-out. You need to send an identify event with unsubscribed_inapp
as true
.
// User level Opt out of in-app messages
// Fire identify event with unsubscribed_inapp as true or false
BlueShift.sharedInstance()?.identifyUser(withDetails: ["unsubscribed_inapp":true], canBatchThisEvent: false)
// User level Opt out of in-app messages
// Fire identify event with unsubscribed_inapp as YES or NO
[[BlueShift sharedInstance] identifyUserWithDetails:@{@"unsubscribed_inapp":@YES} canBatchThisEvent:NO];
Point out Blueshift's Push Notifications
From iOS SDK v2.1.8, If you send push notifications to your customers in addition to the ones from the Blueshift platform, you can use the isBlueshiftPushNotification
helper methods to point out which push notifications come from our platform:
BlueShift.sharedInstance()?.isBlueshiftPushNotification(userInfo)
BlueShift.sharedInstance()?.isBlueshiftPushNotification(notification.request.content.userInfo)
BlueShift.sharedInstance()?.isBlueshiftPushNotification(response.notification.request.content.userInfo)
[[BlueShift sharedInstance] isBlueshiftPushNotification: userInfo];
[[BlueShift sharedInstance] isBlueshiftPushNotification:notification.request.content.userInfo];
[[BlueShift sharedInstance] isBlueshiftPushNotification: response.notification.request.content.userInfo];
Refer this code from our sample application for more details.
Identify Blueshift push, in-app and inbox deep links
SDK v2.1.12
onwards, the options
dictionary of the OpenURL
method shares additional details about the deep link for push and in-app. The details are in the form of a key-value pair dictionary.
Below is the sample dictionary format that you can expect for push and in-app deep links.
Push notifications
["source": "Blueshift", "channel": "push", "userInfo":["alert":"hello"]]
For push notifications, the options dictionary now contains the push notification payload under the key userInfo
.
In-App notifications
["source": "Blueshift", "channel": "inApp", "inAppNotificationType": "modal", "clickedButtonText": "Sure", "clickedButtonIndex": "btn_0"]
SDK v2.4.0
onwards, the options dictionary of the OpenURL
method shares additional details about the inbox message.
Inbox messages
["source": "Blueshift", "channel": "inbox", "inAppNotificationType": "modal", "clickedButtonText": "Sure", "clickedButtonIndex": "btn_0"]
The clickedButtonText
and clickedButtonIndex
are only applicable to modal in-apps.
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey : Any] = [:]) -> Bool {
// Check if deep link url is from Blueshift.
if let source = options[UIApplication.OpenURLOptionsKey(rawValue: "source")] as? String, source == "Blueshift" {
showProductDetail(animated: true, url: url)
}
return true
}
- (BOOL)application:(UIApplication *)app openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
// v2.1.12 - Check if deep link url is from Blueshift.
if([options[@"source"] isEqual: @"Blueshift"]) {
[self showProductDetail:url];
}
return YES;
}
Set custom push notification authorization options and notification categories
From SDK v2.2.0
onwards, you can use the 'config' object in the SDK to set custom push notification authorization options and notification categories, which the SDK will use while registering for the remote notifications. You can set them during SDK initialization.
Set custom authorization options
Set your custom authorization options to the customAuthorizationOptions
property of the config
object during SDK initialization. The given options will be used while registering for the remote notifications.
If you do not set this property during SDK initialization, then SDK will use the default options. The default authorization options used by the SDK are alert
, badge
, and sound
. You can set custom authorization options as follows:
//Set custom authorization options
config.customAuthorizationOptions = [.alert, .badge, .sound, .providesAppNotificationSettings]
//Set custom authorization options
config.customAuthorizationOptions = UNAuthorizationOptionAlert| UNAuthorizationOptionSound| UNAuthorizationOptionBadge| UNAuthorizationStatusProvisional;
Set custom notification categories
Set your custom notification categories to the customCategories
property of the config
object during SDK initialization. The given categories will be merged with the SDK's custom categories and they will be set to the UNUserNotificationCenter
while registering for the remote notifications.
The customCategories
property accepts Set
of UNNotificationCategory
objects. You can set the custom categories as follows. The function getCustomeCategories()
returns a set of UNNotificationCategory
objects.
//Set custom categories
//The method getCustomeCategories() should return set
config.customCategories = getCustomeCategories();
//Set custom categories
config.customCategories = [self getCustomeCategories];
SDK push notification callbacks
The SDK provides callbacks for these events:
- When a user taps on the push notification
- When a user taps on the action button on custom action button push notification
- When a user taps on the carousel push notification image
You can write these callbacks in the AppDelegate
class by implementing the BlueShiftPushDelegate
protocol on the AppDelegate and set the blueShiftPushDelegate
as self
, but if you want to implement callbacks in a separate class, then your app must pass the custom class reference in the BlueShiftConfig
class when the app initializes the SDK.
To pass the custom class reference, create a class that implements the BlueShiftPushDelegate
protocol. For example, create BlueshiftPushNotificationEvents
class to receive the callbacks of push notifications.
You can find here more about the push notification callback methods.
// BlueshiftPushNotificationEvents is the class for handling
// BlueShiftPushDelegate delegate methods
let blueshiftPushDelegate = BlueshiftPushNotificationEvents()
config.blueShiftPushDelegate = blueshiftPushDelegate
// BlueshiftPushNotificationEvents is the class for handling BlueShiftPushDelegate delegate methods
BlueshiftPushNotificationEvents *blueshiftPushDelegate = [[BlueshiftPushNotificationEvents alloc] init];
[config setBlueShiftPushDelegate:blueshiftPushDelegate];
Note
To avoid duplicate deep-link triggers, don't use deep-links in the push notification callbacks from push notification clicks.
Customise the Go to Settings pop-up text
From SDK v2.2.6, You can send in-app notifications with a special deep link blueshift://req-push-permission
, and if a user clicks on the in-app notification button then,
- If push permission is not asked to the user, then SDK will show a push permission dialog.
- If user push permission is already given, then nothing will be displayed after clicking on the in-app notification button.
- If push permission is denied or push notifications are disabled, then the SDK will show a pop-up asking to go to the app settings to enable push notifications. The text of this pop-up is customizable and can be configured using Localizable strings.
Refer to the following screenshot for in-app notification, push permission dialog, and the Go to setting pop-up. The Go to setting pop-up is rendered with default text provided by the SDK in the English language.
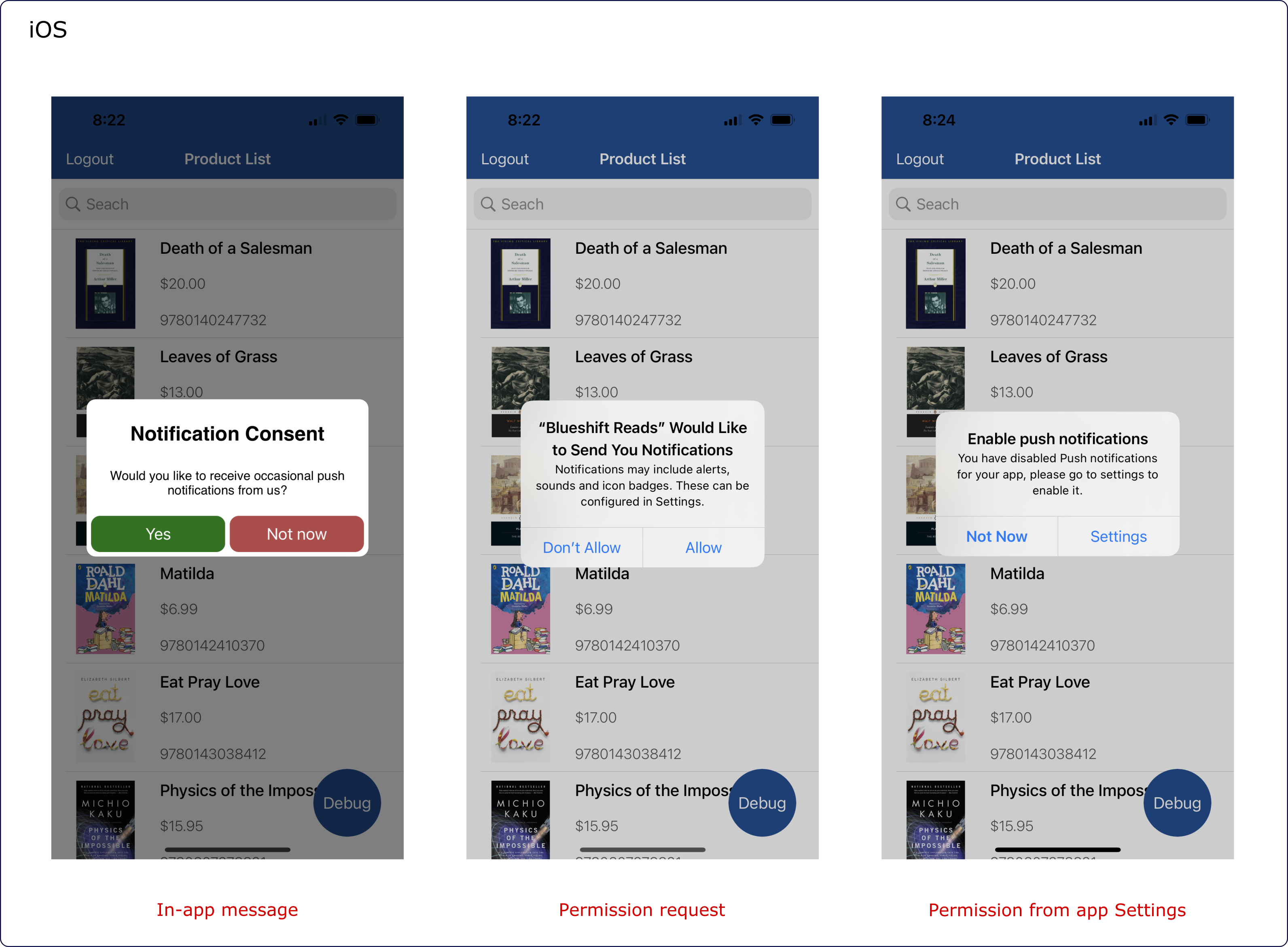
The pop-up text can be customized using Localization. You can add the below keys and your custom localized text in your Localizable.strings
files, and SDK will take care of picking them up based on the app/device language.
Localizable keys -
- BLUESHIFT_GOTOSETTING_ALERT_TITLE
- BLUESHIFT_GOTOSETTING_ALERT_TEXT
- BLUESHIFT_GOTOSETTING_ALERT_OKAY_BUTTON
- BLUESHIFT_GOTOSETTING_ALERT_CANCEL_BUTTON
Refer to our sample app implementation #1 and #2 to know more.
Disable SDK tracking
SDK tracks the custom events, push metrics, and in-app metrics by default. From SDK v2.1.14, you can stop SDK from tracking these events based on your use-case.
Disabling the SDK will stop sending custom events and metrics for push and in-app to Blueshift’s server. It will also remove any pending events (batched or real-time) that are yet to be sent to the server.
/// Disable SDK events tracking
BlueShift.sharedInstance()?.enableTracking(false)
To start the SDK events tracking once again after disabling it, you will need to enable it explicitly by calling
BlueShift.sharedInstance()?.enableTracking(true)
.
Location tracking
From iOS SDK v2.1.7, SDK will not track the user location automatically. The host application needs to set the updated location to the Blueshift SDK in order to track the user location. It is the host app's responsibility to ask location permission and update the latest location to SDK.
//Set updated location to SDK to track the location
BlueShiftDeviceData.current()?.currentLocation = currentLocation
//Set updated location to SDK to track the location
[[BlueShiftDeviceData currentDeviceData] setCurrentLocation: currentLocation]
Refer this code from our sample application for more details.
IDFA tracking
From iOS SDK v2.1.17, SDK will not track the IDFA automatically. The host application needs to set the IDFA value to the Blueshift SDK in order to track the IDFA. It is the host app's responsibility to ask IDFA tracking permission and set the value to SDK. We recommend setting the IDFA value immediately after the app launch. You can set the IDFA to SDK as below.
// Set the IDFA value to SDK if user has accepted the tracking permission
BlueShiftDeviceData.current()?.deviceIDFA = ASIdentifierManager.shared().advertisingIdentifier.uuidString
// Set the IDFA value to SDK if user has accepted the tracking permission
[[BlueShiftDeviceData currentDeviceData] setDeviceIDFA:[ASIdentifierManager sharedManager].advertisingIdentifier.UUIDString];
Opt-out of silent push notification registration
SDK registers for silent push notifications by default in order to send in-app notifications when push permission is not asked the user or push permission is not accepted by the user.
From SDK v2.1.13, you can opt-out from silent push notification registration by setting config.enableSilentPushNotification
to false
.
// Stop SDK from registering for silent(background) push notifications
config.enableSilentPushNotification = false
// Stop SDK from registering for silent(background) push notifications
[config setEnableSilentPushNotification:NO];
Summary
- The SDK is configured to send identify calls.
- The SDK is ready to receive the Push notifications from the Blueshift Dashboard.
Test the SDK integration
These are some of the guidelines to verify the correctness of the SDK integration. The most important thing is to ensure that the app is working as it was before integrating with the SDK.
-
SDK is initialized correctly
Ensure that you do not delay the SDK initialization as it may impact push click tracking. Make sure certain events are launched correctly from the app, such asidentify
orapp_open
. You can confirm that the events show up correctly in the activity of a user on the Blueshift dashboard. -
Push Notifications
Use the push studio editor to create different types of push messages and ensure that the notifications render correctly on devices. This push configuration will enable the basictitle + content
notifications. To configure the Rich push notifications, see this. -
Campaigns
Create a test campaign for push notifications with the test devices and run it against them to ensure that you are gettingdelivered
andclick
stats.
That's it! You are all set to start using the SDK!
Updated about 11 hours ago
Now that you're familiar with our SDK, you can start tracking events on your app. For more information, see: